Suppose we have the following two points in a 3D space:
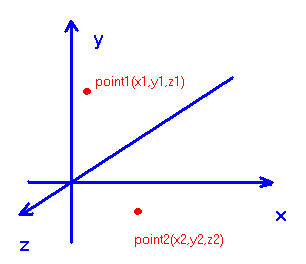
The distance between point1 and point2 is:

Please create ThreeD class inherited from Point class which is used in previous exercise and then use ThreeD class to calculate the distance between two 3D points.
Hint:
- Add two more public functions to Point class to get the value of X and Y
- ThreeD initialize:
-
class ThreeD:public Point
- The following constructor of ThreeD class reuses the constructor of the Point class and the only way values can be passed to the Point constructor is via the use of a member initialization list.
-
ThreeD(double i, double j, double k):Point(i,j){z = k;}
Example input:
1 1 1
2 3 4
Example output:
Distance is: 3.74166