- Create a project.
- Divide the program (progtest.c) into several files. You do it according to each function. For example, progmain.c, area.c, peri.c, and show.c. progmain.c is the main program. It will call area() function in (area.c) and peri() function in (peri.c).
- Write a header file to define macro definitions and prototypes of functions.
- Execute the main program.
- Compress the whole project into a file and upload it.
/* progtest.c, an example of a large program */
#include <stdio.h>
#include <stdlib.h>
#include <math.h> /* math.h */
#define PI 3.1416
double area(double r);
double peri(double r);
void show(double r);
int main(void) /* main() */
{
printf("area(2.2)=%5.2f\n",area(2));
printf("peri(1.4)=%5.2f\n",peri(3));
system("pause");
return 0;
}
double area(double r) /* area(),compute the area of a circle */
{
show(r);
return (PI*pow(r,2.0)); /* pow(r,2.0), compute square of r */
}
double peri(double r) /* peri(),compute periphery */
{
show(r);
return (2*PI*r);
}
void show(double r) /* show(),shwo radius */
{
printf("Radius is %5.2f, ",r);
}
To upload a file:
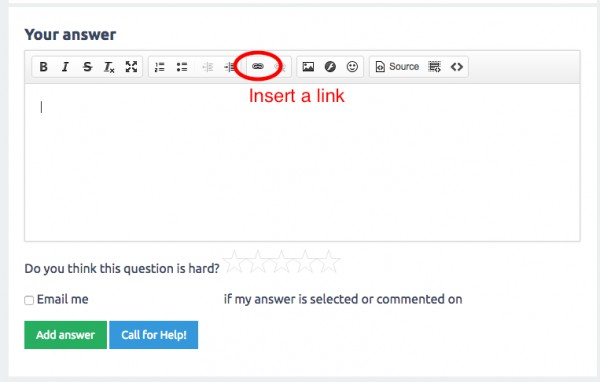
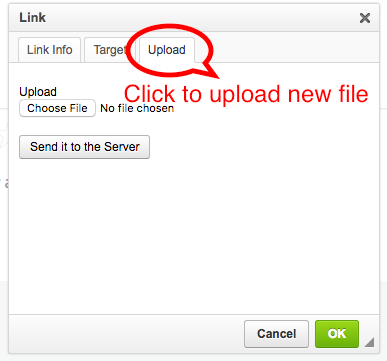
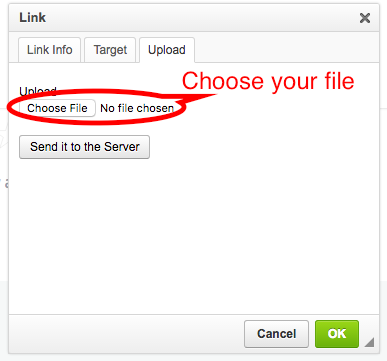
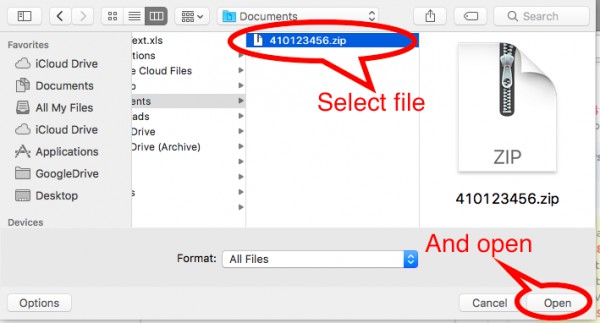
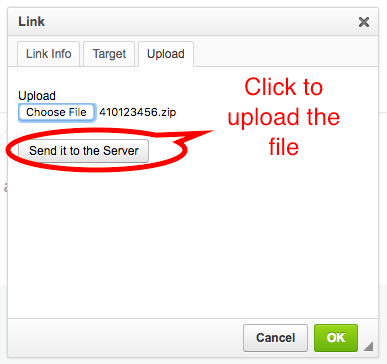
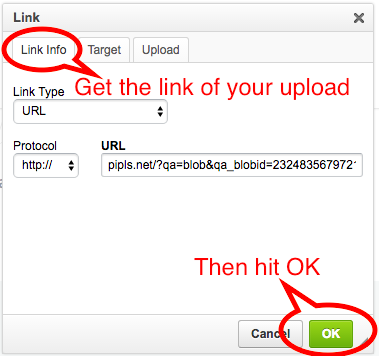