Please write a program receive 2 sets of two-dimensional arrays: a[ ][ ] and b[ ][ ]; size 3*3. Then add a value of each element from a to b for example: a[0][0] + b[0][0], a[0][1] + b[0][1] and so on. Finally, output the summation of each addition.
For more information: http://pipls.net/41053/multi-dimensional-arrays-in-c-language
Input 1
1 2 3
4 5 6
7 8 9
1 1 1
1 1 1
1 1 1
Output 1
1 2 3
4 5 6
7 8 9
1 1 1
1 1 1
1 1 1
2 3 4
5 6 7
8 9 10
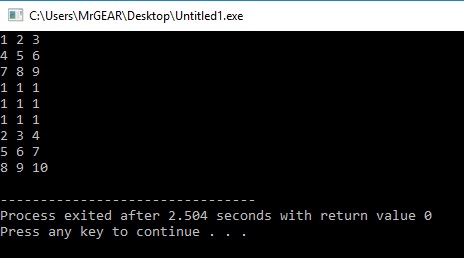
Input 2
1 1 1
0 0 0
1 1 1
2 2 2
2 2 2
2 2 2
Output 2
1 1 1
0 0 0
1 1 1
2 2 2
2 2 2
2 2 2
3 3 3
2 2 2
3 3 3