Please write a program that can identify a value's characteristic based on ASCII table. It must identify:
- 0-9 is a digit
- A-Z is a capital character
- a-z is a small character
- Besides those conditions, it shows '%c is unknown'.
Kindly use the given template to finish the task. Be advised that your outputs must be exactly the same as the shown outputs below.
Example(Template)
#include <stdio.h>
#include <stdlib.h>
/* run this program using the console pauser or add your own getch, system("pause") or input loop */
int main(int argc, char *argv[]) {
char a;
printf("Define:\n");
scanf("%c",&a);
// Your condition starts from here
return 0;
}
Input#1
Define:
6
Output#1
6 is a digit
Input#2
Define:
A
Output#2
A is a capital character
Input#3
Define:
f
Output#3
f is a small character
Input#4
Define:
/
Output#4
/ is unknown
Suggestion
It's time to use your favourite table: ASCII
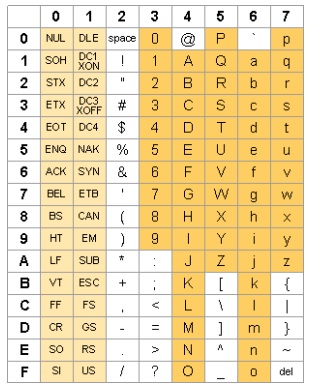