Please write a program that receives 7 characters aweSOME in the same line. It then changes the first 3 lowercase characters into uppercase and the last 4 uppercase characters into lowercase. Kindly use a given template to finish the task.
Example(template)
#include <stdio.h>
#include <stdlib.h>
/* run this program using the console pauser or add your own getch, system("pause") or input loop */
int main(int argc, char *argv[]) {
char s1,s2,s3,s4,s5,s6,s7; // You can change these declarations, it entirely depends on you.
printf("Input your characters:\n"); // Do not change this
// Your conditions start from here
return 0;
}
Input
Input your characters:
aweSOME
Output
AWEsome
Suggestion
You may need a little help from your friendly table, here is ASCII table.
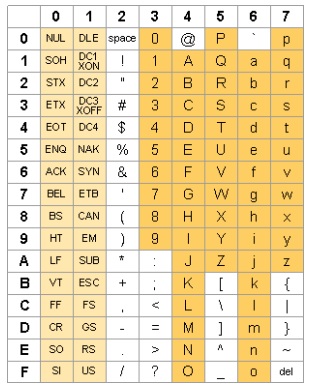